Overview
Clean.Net provides two caching implementations and flexible ways to implement caching in your application.
- Memory Cache: A fast and simple in-memory cache solution that is ideal for applications hosted on a single instance.
- Redis Cache: A distributed cache solution which offers data consistency when hosting multiple application instances, advanced data structures and features
Choose the option that best suits your needs. This can be configured in the
/src/api/appsettings.json
file. If you are using Redis, you need to set up a Redis
server and provide the connection string in the configuration file.
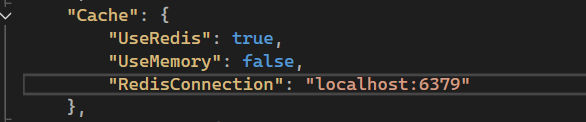
Usage
1. Attribute-based (AOP)
[Cache(cacheKeyPrefix: "key-prefix", durationInSeconds: 60)]
public async Task<Data> GetData(int id)
{
// Method implementation
}
2. Manual Cache Management
public class Service
{
private readonly ICache _cache;
private readonly IRepository _repository;
public Service (ICache cache, IRepository repository)
{
_cache = cache;
_repository = repository;
}
public async Task<Data> GetData(int id)
{
var key = $"data-{id}";
var data = _repository.GetDataById(id);
return await _cache.GetOrSetAsync(key, data);
}
}
3. Cache Eviction
Clean.Net provides cache eviction through:
- Automatic time-based expiration
- Manual eviction using [EvictCache] attribute
- Programmatic eviction via ICache